In order to pass PHP values to a JavaScript file in WordPress you have to make use of the wp_localize_script function. Before you can use the wp_localize_script function to access your localized JavaScript variables, you need to first register the script and then enqueue the script in a PHP file.
We’re using the WordPress Plugin Boilerplate in this example and we created a simple PHP to JavaScript WordPress Plugin repository available at GitHub so that you can see how it works if you run into any issues (this is visible at the bottom of this article).
For the purposes of this example, we’re using the WordPress Plugin Boilerplate (WPPB) framework when building out WordPress plugins. Check out our post introducing working with the WordPress Plugin Boilerplate if you would like to learn more.
Step 1: Register WordPress JavaScript File
You need to open the class-plugin-name-admin.php file in your admin directory. Then, copy the function below and paste it in your enqueue_scripts function
wp_register_script( $this->plugin_name, plugin_dir_url( __FILE__ ) . 'js/plugin-name-admin.js', array(), $this->version, false );
If your script is dependent on jQuery, you would want to adjust the parameters to look like the following:
wp_register_script( $this->plugin_name, plugin_dir_url( __FILE__ ) . 'js/plugin-name-admin.js', array( 'jquery' ), $this->version, false );
Step 2: Enqueue WordPress JavaScript File
Next, you need to run the wp_enqueue_script function in the enqueue_scripts method of the class-plugin-name-admin.php file.
wp_enqueue_script( $this->plugin_name);
Step 3: Create PHP variables to Pass to JavaScript File
Create the add_something_nonce, the ajax_url, and the user_id variables in your enqueue_script method (within the class-plugin-name-admin.php file), because we want to pass the current user’s id, the ajax_url, and the add_something nonce to our JavaScript file.
$add_something_nonce = wp_create_nonce( "add_something" );
$ajax_url = admin_url( 'admin-ajax.php' );
$user_id = get_current_user_id();
These values are used because they have all proved to be valuable in practice when building out WordPress plugins. I have consistently used all three throughout my WordPress plugin development experiences.
Step 4: Run wp_localize_script
We’ve finally reached the meat and potatoes of the post, the wp_localize_script function. We will add this to our enqueue_scripts method. The first parameter has to be associated with the script that you registered above, the plugin_name property in our case; this sets the JavaScript file to pass the variables to. The second parameter sets the name of the JavaScript variable that contains the variable values. The third parameter is an array of variable values to pass to the JavaScript file.
wp_localize_script( $this->plugin_name, 'plugin_name_ajax_object',
array(
'ajax_url' => $ajax_url,
'add_something_nonce'=> $add_something_nonce,
'user_id' => $user_id
)
);
When you combine all of these steps together, your full enqueue_scripts method will look like the following (within the class-plugin-name-admin.php file):
public function enqueue_scripts() {
/**
* This function is provided for demonstration purposes only.
*
* An instance of this class should be passed to the run() function
* defined in Migrate_Loader as all of the hooks are defined
* in that particular class.
*
* The Migrate_Loader will then create the relationship
* between the defined hooks and the functions defined in this
* class.
*/
wp_register_script( $this->plugin_name, plugin_dir_url( __FILE__ ) . 'js/plugin-name-admin.js', array( 'jquery' ), $this->version, false );
wp_enqueue_script( $this->plugin_name);
// Set Nonce Values so that the ajax calls are secure
$add_something_nonce = wp_create_nonce( "add_something" );
$ajax_url = admin_url( 'admin-ajax.php' );
$user_id = get_current_user_id();
// pass ajax object to this javascript file
// Add nonce values to this object so that we can access them in the plugin-name-admin.js javascript file
wp_localize_script( $this->plugin_name, 'plugin_name_ajax_object',
array(
'ajax_url' => $ajax_url,
'add_something_nonce'=> $add_something_nonce,
'user_id' => $user_id
)
);
}
Step 5: Access Localized Variables in JavaScript File
Open up your plugin-name-admin.js file in your admin/js directory. In this file, you can see the localized variable values with the following code:
(function( $ ) {
'use strict';
console.log(plugin_name_ajax_object);
console.log(plugin_name_ajax_object.ajax_url);
console.log(plugin_name_ajax_object.user_id);
console.log(plugin_name_ajax_object.add_something_nonce);
})( jQuery );
If you open up your web browser and navigate around your WordPress admin dashboard, inspect the page by right-clicking on the page and click on Inspect (or Inspect Element).
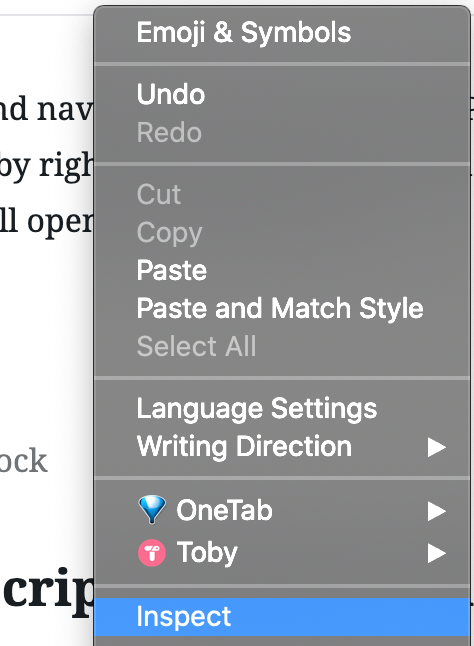
This will open up your inspector. Click on the Console tab in the Inspector and reload the page.
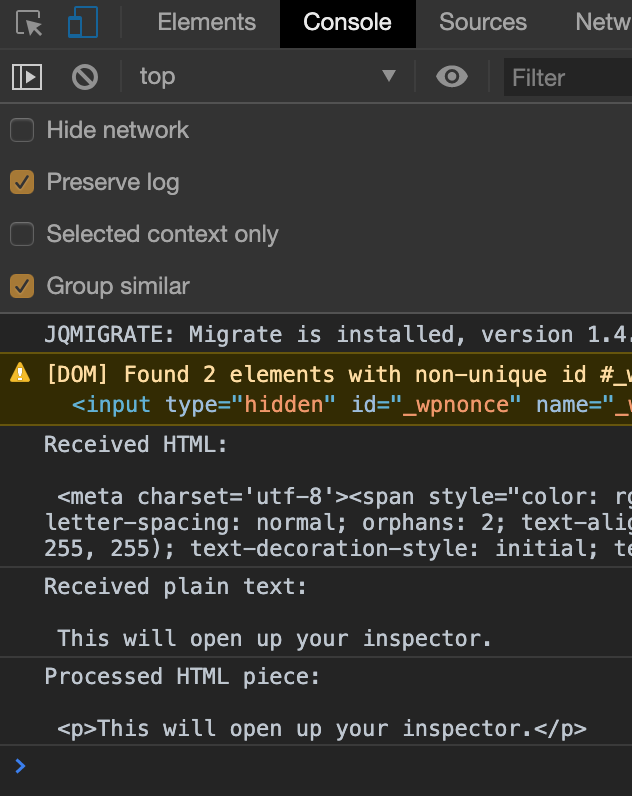
If you don’t see the values in the console, reload the page again and you should see the different values that were added to the console log in the JavaScript function above.
The Pass PHP to JavaScript WordPress Plugin GitHub Repository
We created this repository for anyone who is having issues with our instructions or just wants to see where the code fits in a WordPress plugin. In our instructions above, we show you how to pass PHP values to the admin JavaScript file but in the repository, we also show you how to pass PHP values to the public JavaScript file, giving a developer more flexibility in the types of functionality they can build in their plugin.