Starting at the big picture level, web development includes what you see when you visit a website and what you can do on that website. The “what you see” part of a website, or its layout and design, are typically built by frontend web developers. The “what you can do” part of a website, or its functionality, interaction with other services, and storage of data in a database tends to be built by backend web developers.
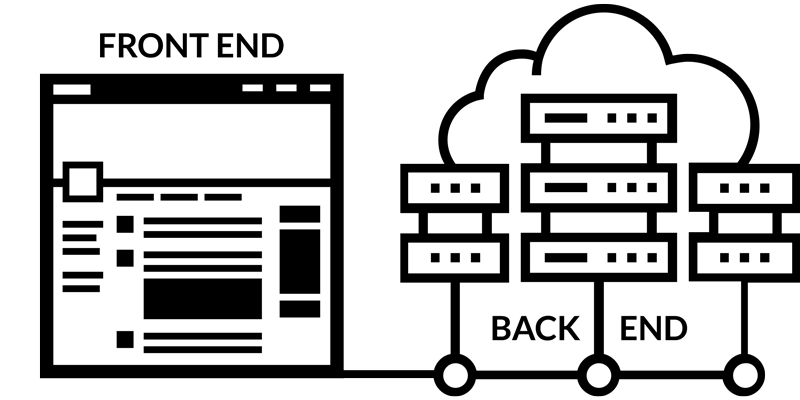
Both the front end and the backend code are stored in a computer or computers, also known as servers, that are connected to the internet. The internet is a series of fiber optic cables that connect these servers to each other. Each server typically has an operating system (usually Linux – which makes it easy to add software to the server) along with a web server (typically Apache or NGINX) which allow the server to rapidly send content to a web browser through the internet. A web browser then interprets the raw code that is sent to it from the server and translates it into the user interface that you see when you visit a website. A server could contain both frontend and backend code or they could have one server dedicated to providing frontend code and send requests to the backend server to complete requested actions.
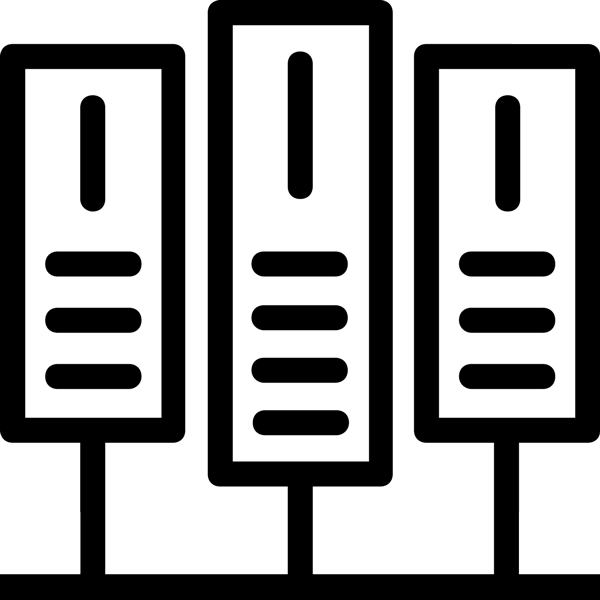
Facebook as a Case Study
If you go to Facebook.com and you are logged out, you will likely see the following page:
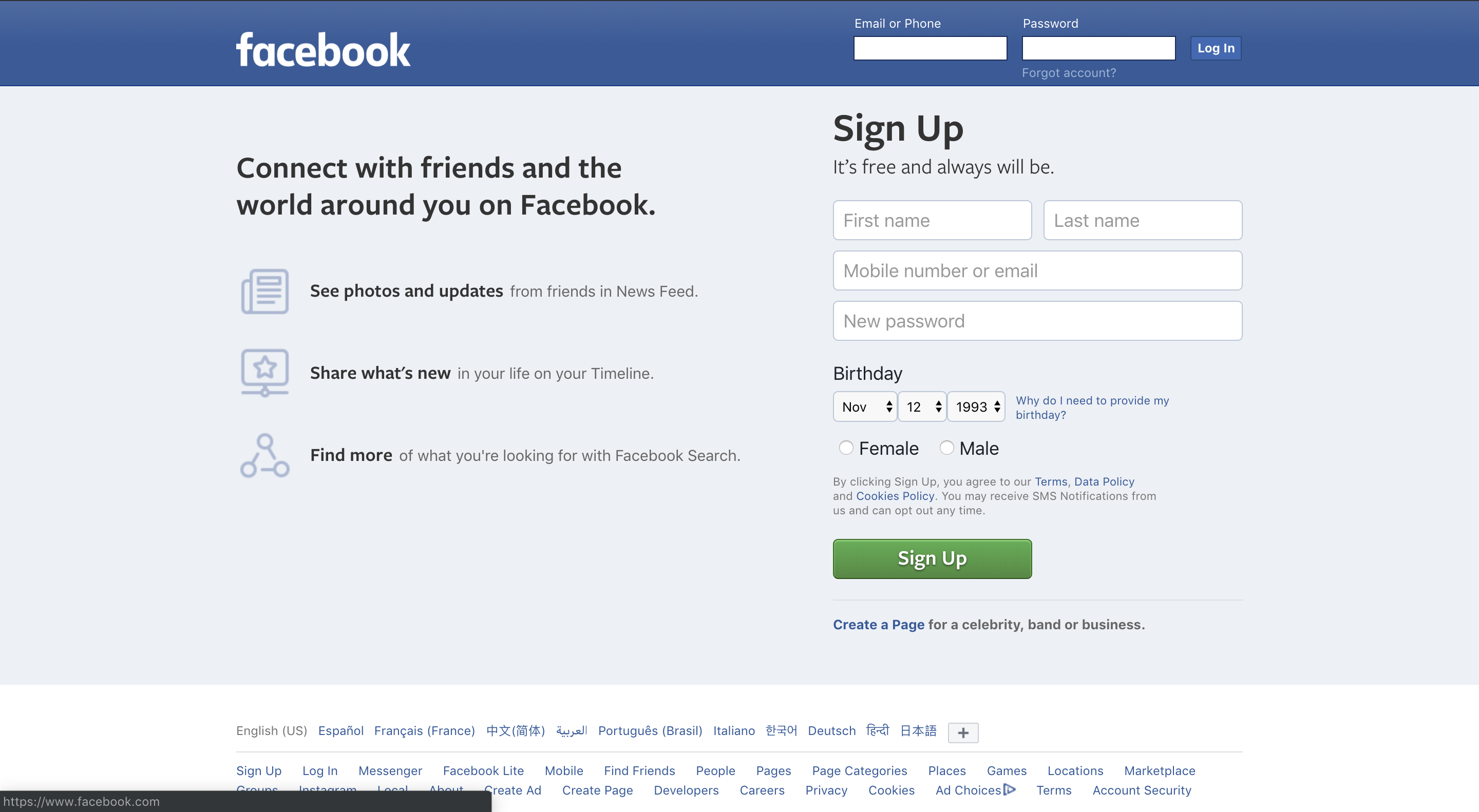
What are the Front End Aspects of this page?
1. The structure of the page: You’ll notice that you have three rows on this page. This is built using HTML. Hyper Text Markup Language contains a number of elements that when added to a page give it it’s structure or layout.
The header: typically the first row (could be the first column on the page as some pages have sidebar headers)
The body or content area: typically the second row
The footer: typically the last row (which contains important links to resources or other pages)
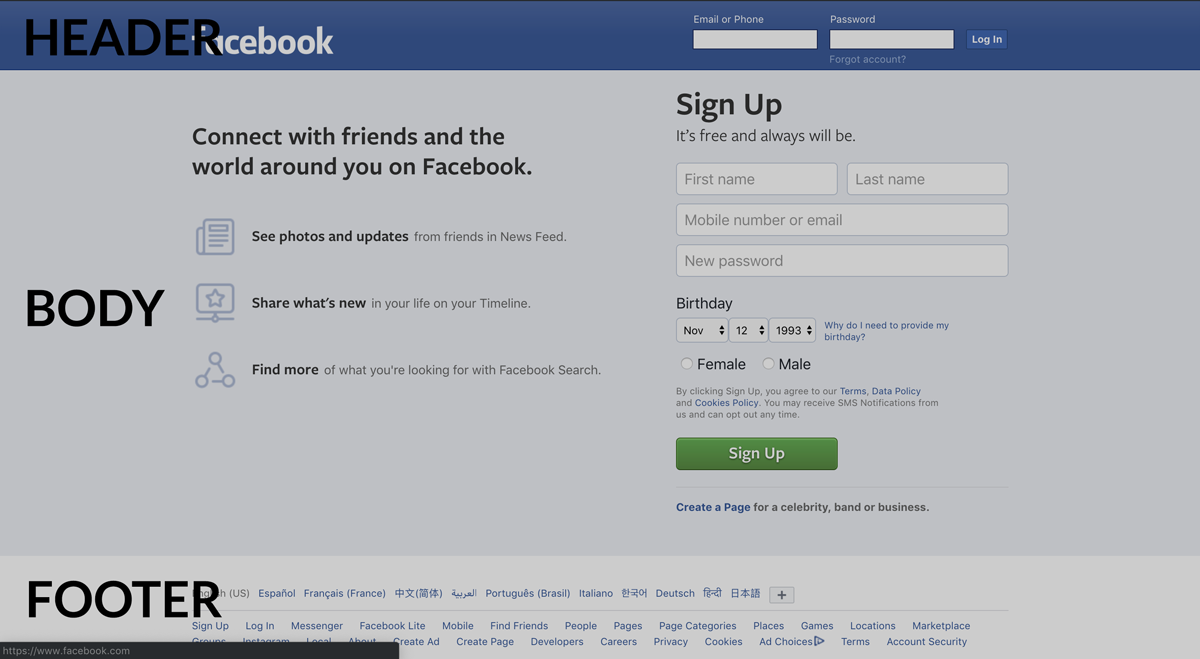
Here’s what the HTML for a very basic web page can look like:
<!DOCTYPE html>
<html>
<head>
<title>The Page Title</title>
</head>
<body>
<h1>The First Heading</h1>
<h2>The Second Heading</h2>
<p>The first paragraph.</p>
</body>
</html>
2. The style of the page: You’ll notice that the background color of the header (the first row) is a dark blue, the background color of the content area (the 2nd row) is a lighter shade of blue, and the background color of the footer (the 3rd row) is white. There are a number of other stylistic elements on this page, such as the Facebook logo, the color, size, and font of the text in the header, the color, size, and font of the text in the body and the color, size, and font of the text in the footer. These stylistic elements are defined in the CSS. CSS stands for Cascading Style Sheets and these style sheets allow you to design a page to allow for all of the styles described above. They allow you to make a different row a different
Here’s what the CSS for a very basic web page can look like:
h1 {
text-align: center;
color: red;
}
h2 {
text-align: center;
color: red;
}
p {
text-align: center;
color: red;
}
What are the Backend Aspects of this page?
In differentiating frontend from backend web development, think about what kinds of actions you can take on that facebook homepage. You can login to an existing account and you can create a new account.
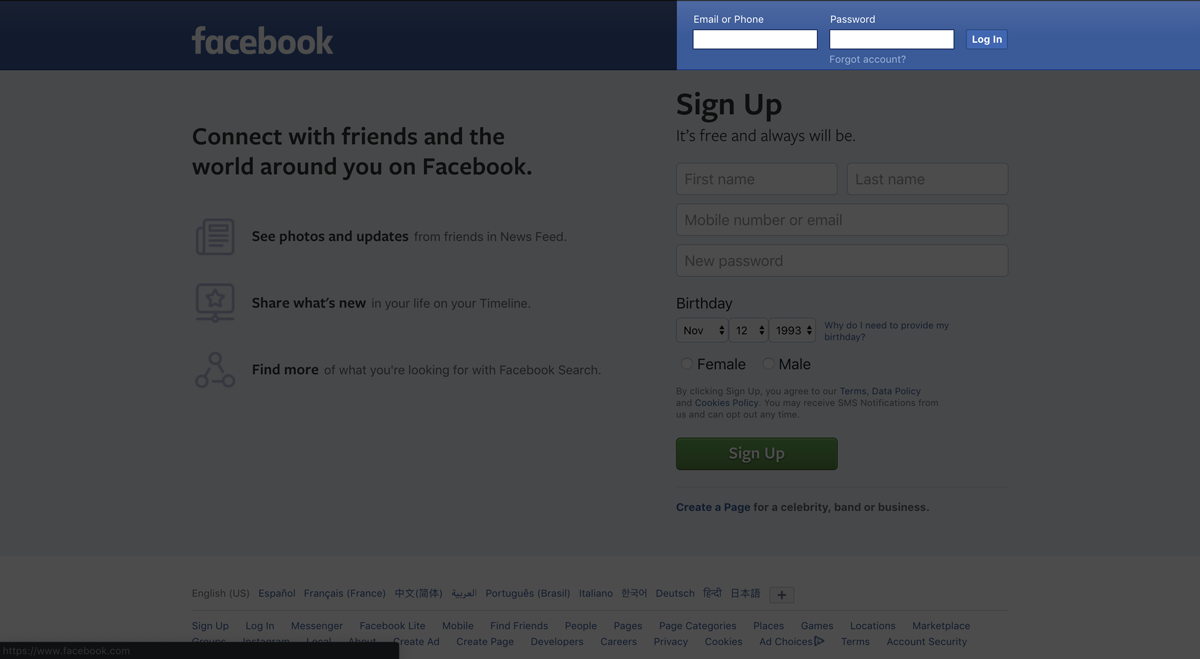
The HTML input elements for the login form and the way they are styled on the homepage are governed by the frontend, but once you enter in your username and password and click on the Log In button, you are initiating an action. In this action, the entered text is sent to one of Facebook’s servers. The data are sent using something called an HTTP request. HTTP stands for HyperText Transfer Protocol and these requests are a central part of the internet. HTTP requests typically fall into 4 categories (commonly referred to as CRUD):
- Create
- Retrieve
- Update
- Delete
So, your username and password are sent via an HTTP request to a server, and, using a backend coding language, your database is scanned to see if the username and password are associated with an existing record in a database. A commonly used database in web development is MySQL. MySQL is so named because “My”, is the name of co-founder Michael Widenius’s daughter, and “SQL”, is the abbreviation for Structured Query Language. A MySQL database consists of databases and tables. You can think of a database as the filing cabinet and the individual drawers as the tables. The papers that you have in the drawers are the records within the table.
If Facebook finds the username and password in their database table, Facebook successfully logs you in and sends you to your news feed page to show you posts that your friends have posted. A commonly used backend language is PHP. PHP stands for Hypertext Preprocessor. You would write a PHP script to connect to the database, to clean the data that you received from the user, to encrypt the password, to check in the correct table to see if the username and password exist, and if it does exist, then you send the user to the dashboard and you create a session to remember them in the future and if it doesn’t exist, you display the error and ask the user to enter their credentials again.
Here’s what the PHP for a very basic login script can look like:
<?php
session_start();
include("connection.php"); //Include a file that is used to connect to our MySQL database
$error = ""; //Variable used for storing errors.
if(isset($_POST["submit"])){
if(empty($_POST["username"]) || empty($_POST["password"])){
$error = "Both fields are required.";
} else {
// Retrieve username and password values and set them equal to their respective variables
$username=$_POST['username'];
$password=$_POST['password'];
// To protect from MySQL injection use stripslashes and real escape string
$username = stripslashes($username);
$password = stripslashes($password);
$username = mysqli_real_escape_string($db, $username);
$password = mysqli_real_escape_string($db, $password);
$password = md5($password);
//Check username and password from users table in the database
$sql="SELECT id FROM users WHERE username='$username' and password='$password'";
$result=mysqli_query($db,$sql);
$row=mysqli_fetch_array($result,MYSQLI_ASSOC);
//If username and password exist in our database then create a session and redirect user to dashboard
//Otherwise display error.
if(mysqli_num_rows($result) == 1){
$_SESSION['username'] = $login_user; // Creating Session
header("location: dashboard.php"); // Redirecting To Dashboard
} else {
$error = "Incorrect username or password.";
}
We tend to use frameworks for our HTML, CSS, and